In the ever-evolving world of technology, Java continues to hold its place as one of the most popular programming languages, powering millions of applications worldwide.
Whether you’re a budding beginner or a seasoned professional, staying ahead in this industry requires mastering a mix of Java developer skills, both foundational and advanced.
In this guide, we’ll delve into the core competencies and cutting-edge tools you need to thrive as a Java developer in today’s competitive market.
Core Java developer skills
Java Syntax and Basics
Java's syntax serves as the bedrock of coding mastery. It’s about understanding how to write clean, precise instructions for the Java Virtual Machine (JVM). This includes:
- Data Types & Variables: Knowing when to use
int
,float
, orString
—the building blocks of Java logic. - Control Structures: Mastering
if-else
,for
, andwhile
loops to control program flow. - Object-Oriented Concepts: Grasping classes, objects, inheritance, and polymorphism—the heart of Java’s design philosophy.
- Exception Handling: Learning to anticipate and manage errors using try, catch, and finally blocks for robust programs.
Whether you're creating simple calculators or multi-functional programs, Java basics provide the tools to make it happen.
Core Java APIs
The Core Java APIs are like Swiss Army knives for developers. They offer pre-built tools to:
- Manipulate Data: With libraries like
java.util
for collections such asArrayList
,HashMap
, and more. - Handle Errors Gracefully: Use
try-catch
blocks from thejava.lang
package to manage exceptions efficiently. - Streamline I/O: The
java.io
andjava.nio
packages enable reading and writing files with ease.
Harnessing these APIs not only saves time but ensures your code is robust and professional.
Databases and JDBC
Java Database Connectivity (JDBC) bridges your application with powerful databases like MySQL, PostgreSQL, or Oracle.
- SQL Integration: Craft queries to insert, update, or retrieve data seamlessly.
- Connection Management: Utilize the
DriverManager
class to create secure and efficient database connections. - Result Processing: Employ
ResultSet
to process and display data dynamically within your app. - Prepared Statements: Enhance security and performance by using PreparedStatement to execute parameterized queries.
In the world of data-driven applications, JDBC is indispensable for turning raw data into actionable insights.
Algorithm Java developer Skills
Algorithms are the secret sauce of a good developer. With a strong grasp of algorithms, you can:
- Optimize Performance: From sorting (
QuickSort
,MergeSort
) to searching (Binary Search
), algorithms make your code faster and smarter. - Tackle Challenges: Solve real-world problems like scheduling tasks, route planning, or data compression.
- Think Logically: Learning to design algorithms enhances your ability to approach problems from multiple angles.
- Analyze Complexity: Evaluate time and space efficiency using Big O notation to write scalable code.
Every efficient application is backed by a solid understanding of algorithms—it's where creativity meets logic.
Create your professional Resume in 10 minutes for FREE
Build My Resume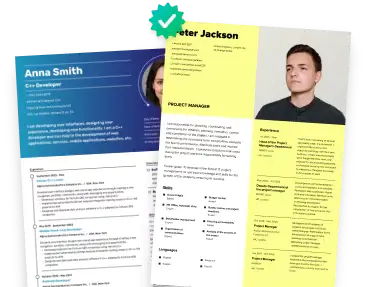
Java backend developer skills
1. Java Core Proficiency
A solid foundation in Java includes understanding object-oriented programming (OOP), exception handling, generics, collections, and multithreading.
- Learning Time: 3-6 months depending on prior programming experience.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Platforms like Codecademy, Udemy, or the book Head First Java. Practice with coding challenges on HackerRank or LeetCode.
2. Spring Framework
The Spring Framework simplifies the development of Java-based applications. Spring Boot allows for rapid development, Spring MVC handles web layers, and Spring Data provides support for database operations. Spring is widely used for creating enterprise-level applications and microservices.
- Learning Time: 4-8 weeks for the basics; 3-6 months to understand deep integrations and microservices.
- Complexity: Intermediate to Advanced
- How/Where to Learn: Books like Spring in Action, or online courses focused on Spring Boot and the Spring Framework.
3. Database Management
A Java backend developer must be proficient in both relational and NoSQL databases. This includes the ability to design and interact with databases, write SQL queries, and understand the trade-offs between using relational (e.g., MySQL, PostgreSQL) and NoSQL (e.g., MongoDB) databases.
- Learning Time: 4-8 weeks for basic CRUD operations; 3-6 months to master advanced concepts like joins, indexing, and performance tuning.
- Complexity: Beginner to Intermediate
- How/Where to Learn: SQL tutorials, MongoDB University (for NoSQL), and practice with databases.
4. API Development
API development skills are crucial for designing RESTful web services using Java. You’ll need to work with HTTP methods (GET, POST, PUT, DELETE), status codes, serialization (JSON/XML), and integration with frontend or third-party services.
- Learning Time: 2-4 weeks for basic API design; 2-3 months for advanced topics such as API security, versioning, and performance tuning.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Books on RESTful web services, API design best practices, and API testing tools.
5. Microservices Architecture
Microservices architecture involves breaking down an application into smaller, independent services that can be developed, deployed, and scaled individually. This approach requires familiarity with discovery, load balancing, and inter-service communication protocols.
- Learning Time: 3-6 months for a solid understanding and hands-on experience.
- Complexity: Advanced
- How/Where to Learn: Books like Building Microservices by Sam Newman, and courses focused on microservices with Spring Boot.
6. Version Control (Git)
Version control systems like Git track in the codebase, enabling collaborative work among teams, rollback of changes, and more structured code management. Understanding branching, merging, and resolving conflicts is crucial for any backend developer.
- Learning Time: 1-2 weeks for the basics; 2-3 months for advanced Git workflows.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Tutorials on Git, Pro Git (book), and GitHub documentation.
7. Performance Optimization
Performance optimization involves improving the speed, scalability, and memory usage of applications. Techniques include profiling, optimizing algorithms, using caching mechanisms and database query performance.
- Learning Time: 4-8 weeks for understanding tools and techniques; 6+ months for mastering optimization strategies.
- Complexity: Advanced
- How/Where to Learn: Books like Java Performance, using profiling tools such as VisualVM, and taking advanced courses.
8. Security
Ensuring application security is crucial to prevent unauthorized access, data breaches, and other vulnerabilities. Common practices include implementing OAuth2, JWT tokens, and ensuring data is encrypted both at rest and in transit.
- Learning Time: 4-8 weeks for basic knowledge; 3-6 months to fully integrate security in development processes.
- Complexity: Intermediate to Advanced
- How/Where to Learn: Security best practices guides like OWASP Top Ten, Spring Security documentation, and security courses.
9. Build Tools (Maven/Gradle)
Build tools like Maven or Gradle are used to automate the building, testing, and deployment of applications. These tools manage dependencies, plugins, and the entire lifecycle of a Java project.
- Learning Time: 1-2 weeks for basics; 2-3 months for integrating in real projects.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Documentation for Maven and Gradle, and online tutorials for practical implementations.
10. Cloud Integration (AWS, Azure, GCP)
Platforms like AWS, Google Cloud, and Azure provide tools for hosting applications, databases, and managing infrastructure. As a backend developer, understanding how to manage and deploy apps in the cloud is a key skill.
- Learning Time: 4-8 weeks for basic deployments; 3-6 months for proficiency in cloud infrastructure management.
- Complexity: Intermediate to Advanced
- How/Where to Learn: Cloud provider training resources and courses focused on services and deployment.
Examples of Java backend skills in resumes:
Java frontend developer skills
1. HTML/CSS
HTML (HyperText Markup Language) is the standard language for structuring web content, and CSS (Cascading Style Sheets) is used for styling and layout. A solid understanding of both is essential for building responsive, user-friendly web pages.
- Learning Time: 2-4 weeks for the basics; 2-3 months for advanced styling techniques like Flexbox and CSS Grid.
- How/Where to Learn: Online tutorials, books on HTML and CSS, and coding practice platforms.
2. JavaScript
JavaScript is the scripting language that enables dynamic behavior on web pages. A frontend developer needs to master DOM manipulation, event handling, and working with asynchronous JavaScript (AJAX, promises, etc.).
- Learning Time: 3-6 months depending on prior experience.
- How/Where to Learn: JavaScript documentation, online coding challenges, and interactive courses.
3. Frontend Frameworks (React, Angular, Vue.js)
Modern JavaScript frameworks like React, Angular, and Vue.js provide powerful tools for building complex web applications. A frontend developer should be proficient in at least one of these frameworks to develop dynamic user interfaces efficiently.
- Learning Time: 4-6 weeks for basic familiarity; 3-6 months for mastering one of the frameworks.
- How/Where to Learn: Documentation for React, Angular, or Vue.js, along with project-based learning resources.
4. Responsive Web Design
Responsive design ensures that web applications are accessible and user-friendly on a variety of devices, including mobile phones, tablets, and desktops. This requires knowledge of CSS media queries, flexible grid systems, and adaptive images.
- Learning Time: 3-6 weeks for the basics; 2-3 months for mastering advanced concepts.
- How/Where to Learn: Online resources, tutorials, and courses on creating responsive layouts using Flexbox, Grid, and media queries.
5. Version Control (Git)
Version control with Git is essential for managing source code, especially when working in a team. It allows developers to track changes, manage branches, and collaborate on projects without overwriting each other's work.
- Learning Time: 1-2 weeks for the basics; 2-3 months for mastering advanced Git workflows.
- How/Where to Learn: Git tutorials, books like Pro Git, and practicing with real-world repositories.
6. Browser Developer Tools
Every modern browser comes with developer tools that help developers inspect HTML, CSS, and JavaScript in real time. Mastery of these tools is essential for debugging and optimizing frontend code.
- Learning Time: 1-2 weeks for basic usage; 2-3 months for advanced debugging and performance analysis.
- How/Where to Learn: Browser documentation, and tutorials on using developer tools in Chrome, Firefox, or Edge.
7. JavaScript ES6+ Features
JavaScript ES6 and later versions introduced many important features such as arrow functions, async/await, destructuring, modules, and classes. These modern features make JavaScript code more concise and maintainable.
- Learning Time: 3-4 weeks for the basics; 1-2 months to fully integrate advanced features in projects.
- How/Where to Learn: JavaScript documentation and tutorials on ES6+ syntax and features.
8. CSS Preprocessors (Sass, LESS)
CSS preprocessors like Sass and LESS allow developers to write more maintainable and modular CSS. They introduce features like variables, nesting, and mixins, making CSS code easier to manage for larger projects.
- Learning Time: 2-4 weeks for basic features; 1-2 months for integrating preprocessors into projects.
- How/Where to Learn: Documentation on Sass or LESS, and tutorials on using them in real-world projects.
9. Testing (Unit, UI)
Testing is an important part of frontend development. Unit testing ensures individual components work as expected, while UI checks the behavior and interaction of elements in the browser. Tools like Jest, Mocha, and Cypress are commonly used.
- Learning Time: 4-6 weeks for the basics; 2-3 months for mastering testing frameworks and best practices.
- How/Where to Learn: Testing libraries' documentation, and courses focused on JavaScript methodologies.
10. Build Tools (Webpack, Babel)
Frontend build tools like Webpack and Babel help in bundling files, transpiling modern JavaScript to be browser-compatible, and optimizing assets for faster load times. Understanding these tools is key for modern frontend development workflows.
- Learning Time: 3-6 weeks for the basics; 2-3 months for advanced configurations and optimizations.
- How/Where to Learn: Official Webpack and Babel documentation, and courses focused on frontend build automation.
Skills for frontend Java developers in a resume:
Full stack Java developer skills
1. Java Core Proficiency
A solid foundation in Java programming includes understanding object-oriented programming (OOP), exception handling, generics, collections, and multithreading.
- Learning Time: 3-6 months depending on your prior programming experience.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Platforms like Codecademy, Udemy, or the book Head First Java. Practice with coding challenges on LeetCode or HackerRank.
2. Frontend Technologies (HTML, CSS, JavaScript)
A Full Stack Developer needs a solid grasp of frontend technologies such as HTML for structure, CSS for styling, and JavaScript for interactivity. These are the building blocks of user interfaces that interact with backend services.
- Learning Time: 3-6 months for a full understanding of frontend concepts.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Online tutorials, books on HTML, CSS, JavaScript, and coding practice platforms.
3. Backend Frameworks (Spring, Node.js)
A Full Stack Developer should be proficient in backend frameworks like Spring for Java or Node.js for JavaScript. These frameworks handle the server-side logic, database connectivity, and API creation.
- Learning Time: 3-6 months for Spring; 4-8 weeks for Node.js.
- Complexity: Intermediate to Advanced
- How/Where to Learn: Online tutorials, books like Spring in Action, and courses focused on backend development with Spring or Node.js.
4. Database Management (SQL & NoSQL)
Understanding both relational databases (like MySQL or PostgreSQL) and NoSQL (like MongoDB) is key. Full Stack Developers need to interact with these databases, write SQL queries, and understand data modeling.
- Learning Time: 4-8 weeks for basic CRUD operations; 3-6 months for mastering database performance and scaling.
- Complexity: Beginner to Intermediate
- How/Where to Learn: SQL tutorials, MongoDB University, and courses that focus on database design and performance.
5. RESTful API Development
Full Stack Developers often build and consume APIs to allow communication between the frontend and backend. Understanding RESTful principles, HTTP methods, status codes, and API security is essential.
- Learning Time: 3-6 weeks for the basics; 2-3 months for advanced API development.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Books on RESTful web services, API documentation, and online API design courses.
6. Cloud Integration (AWS, Azure, GCP)
Understanding how to deploy and manage applications in the cloud is a critical high income skill for Full Stack Developers. This includes using services for hosting, storage, and monitoring.
- Learning Time: 4-8 weeks for basic cloud deployments; 3-6 months for proficiency in infrastructure management.
- Complexity: Intermediate to Advanced
- How/Where to Learn: Cloud provider training resources, hands-on labs, and courses focused on cloud services and deployment.
7. Version Control (Git)
Version control is crucial for any developer, particularly when working on teams. Git allows Full Stack Developers to collaborate efficiently, manage codebase history, and track changes.
- Learning Time: 1-2 weeks for the basics; 2-3 months for mastering Git workflows.
- Complexity: Beginner to Intermediate
- How/Where to Learn: Git tutorials, books like Pro Git, and practicing with real-world projects on GitHub.
8. Testing (Unit, Integration, UI Testing)
Full Stack Developers should know how to write tests for both the frontend and backend. This includes unit tests for business logic, integration tests for API interactions, and UI tests for user-facing components.
- Learning Time: 4-8 weeks for basic testing frameworks; 3-6 months to master practices for both frontend and backend.
- Complexity: Intermediate
- How/Where to Learn: Testing libraries' documentation (Jest, Mocha, JUnit), and online courses on test-driven development.
9. DevOps and Continuous Integration/Continuous Deployment (CI/CD)
DevOps principles and CI/CD pipelines help automate testing, deployment, and delivery of software. Full Stack Developers should understand these concepts to streamline development and deployment workflows.
- Learning Time: 2-3 months for the basics; 6+ to become proficient with CI/CD tools like Jenkins, Docker, and Kubernetes.
- Complexity: Advanced
- How/Where to Learn: DevOps tutorials, courses on Jenkins and Docker, and online resources for Kubernetes.
10. Problem-Solving and Algorithmic Thinking
As a Full Stack Developer, strong problem-solving skills are necessary to approach both frontend and backend challenges. This involves algorithm design, data structures, and understanding of complexity.
- Learning Time: 3-6 months to develop strong algorithmic skills; 6+ for advanced problem-solving.
- Complexity: Intermediate to Advanced
- How/Where to Learn: Coding platforms like HackerRank, LeetCode, or books on algorithms and problem-solving.
Java full stack developer skills in resume examples:
Conclusion
Mastering skills for a Java developer is about more than just understanding the language’s syntax. It’s about embracing continuous learning, staying updated with industry trends, and refining problem-solving abilities.
By building expertise in areas like Java frameworks, database integration, and modern deployment practices, you position yourself as a sought-after professional in a dynamic job market.
Start cultivating these competencies today to unlock opportunities and achieve lasting success in your career.
Create your professional Resume in 10 minutes for FREE
Build My Resume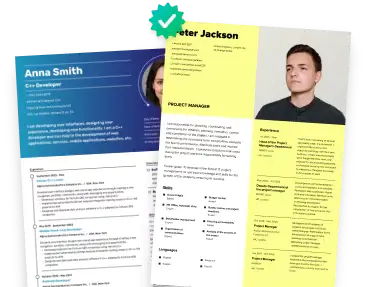